Laravel 11 has been released, requiring PHP v8.2 at minimum. It brings significant enhancements, including a new Laravel Reverb package for real-time capabilities, a streamlined directory structure, per-second rate limiting, health routing, encryption key rotation, queue testing improvements, Resend mail transport, Prompt validator integration, and new Artisan commands. Notably, Laravel Reverb introduces a first-party, scalable WebSocket server for robust real-time functionality in applications.
Requirements for Laravel 11:
Laravel 11.x requires a minimum PHP version of 8.2.
Streamlined Application Structure
The Application Bootstrap File
Laravel 11 brings a significant change with a more minimalistic application skeleton. This involves removing specific folders such as app/Console, app/Exceptions, and app/Http/Middleware. Additionally, the routes folder has been streamlined. The files routes/channel.php, routes/console.php, and routes/api.php have been eliminated.
Note: These structure changes are optional. They will only be the default for new projects, while older Laravel applications can retain the old structure if desired.
Laravel 11 New Slim Skeleton:
app/
├── Http/
│ └── Controllers/
│ └── Controller.php
├── Models/
│ └── User.php
└── Providers/
└── AppServiceProvider.php
bootstrap/
├── app.php
└── providers.php
config
...
Routes, Middlewares, and Exceptions are now registered in the bootstrap/app.php file.
use Illuminate\Foundation\Application;
use Illuminate\Foundation\Configuration\Exceptions;
use Illuminate\Foundation\Configuration\Middleware;
return Application::configure(basePath: dirname(__DIR__))
->withRouting(
web: __DIR__.'/../routes/web.php',
commands: __DIR__.'/../routes/console.php',
health: '/up',
)
->withMiddleware(function (Middleware $middleware) {
//
})
->withExceptions(function (Exceptions $exceptions) {
//
})->create();
Service Provider
In Laravel 11, the default application structure simplifies from five service providers to a single AppServiceProvider. The functionalities of the removed service providers are now managed either automatically by the framework or integrated into the bootstrap/app.php file.
For instance, event discovery is now enabled by default, reducing the need for manual event and listener registration. However, if manual registration is necessary, it can be done in the AppServiceProvider.
Similarly, functionalities like route model bindings or authorization gates previously handled by separate providers can now be registered within the AppServiceProvider.
Opt-in API and Broadcast Routing
In Laravel 11, the default application no longer includes api.php and channels.php route files. Developers can easily create them using Artisan commands if needed.
php artisan install:api
php artisan install:broadcasting
No more Middleware hence no Http/Kernel
In earlier Laravel versions, new applications came with nine middleware handling tasks like request authentication. In Laravel 11, these middleware are integrated into the framework to streamline application structure. Customization methods for them are accessible from bootstrap/app.php.
Most of the things you used to could do in the Kernel you can now do in the Bootstrap/App.
->withMiddleware(function (Middleware $middleware) {
$middleware->validateCsrfTokens(
except: ['stripe/*']
);
$middleware->web(append: [
EnsureUserIsSubscribed::class,
])
})
New Schedule Facade
In Laravel 11, scheduled tasks can now be defined directly in the application’s routes/console.php file using the new Schedule facade, eliminating the need for a separate console “kernel” class.
use Illuminate\Support\Facades\Schedule;
Schedule::command('emails:send')->daily();
Exception Handling in app.php
In Laravel 11, exception handling customization is now done from bootstrap/app.php, along with routing and middleware setup, simplifying application structure.
->withExceptions(function (Exceptions $exceptions) {
$exceptions->dontReport(MissedFlightException::class);
$exceptions->report(function (InvalidOrderException $e) {
// ...
});
})
New Default Database
In Laravel 11 the default database has been changed to SQLite. It will require SQLite 3.35.0 or greater if you choose to use SQLite.
Route Changes
Laravel 11 introduces streamlined handling for API routes and websocket broadcasting. The routes/api.php file and Sanctum package are not included by default. To set up API scaffolding and install Sanctum, you can utilize the php artisan install:api
command.
// Command to install API scaffolding and Sanctum
php artisan install:api
Running this command creates the routes/api.php file and registers it in the bootstrap/app.php. Additionally, Laravel Sanctum will be installed. To enable Sanctum’s functionality, you only need to add the Laravel\Sanctum\HasApiTokens
trait to the User Model.
// Add Laravel Sanctum trait to User Model
use Laravel\Sanctum\HasApiTokens;
class User extends Authenticatable
{
use HasApiTokens;
}
Similarly, websocket broadcasting is made installable. You can prepare your application for broadcasting by using the php artisan install:broadcast
command.
New up Health Route
In Laravel 11, a new /up
health route will be added to facilitate better integration with uptime monitoring, triggering a DiagnosingHealthEvent
.

Limit Eager Load
In Laravel 11, native support for limiting eagerly loaded records will be introduced, eliminating the need for external packages.
User::select('id', 'name')->with([
'articles' => fn($query) => $query->limit(5)
])->get();
New once() method
Laravel 11 introduces a new once
() helper method, ensuring consistent return values for object methods, useful for one-time code execution.
function random(): int
{
return once(function () {
return random_int(1, 1000);
});
}
random(); // 123
random(); // 123 (cached result)
random(); // 123 (cached result)
Casts Method
In Laravel 11, casts will now be defined within the protected method casts()
instead of the $casts
property.
class User extends Authenticatable
{
// ...
protected function casts(): array
{
return [
'email_verified_at' => 'datetime',
'password' => 'hashed',
];
}
}
APP_KEY Rotation
Changing encryption key in Laravel used to log out users and break old data. Laravel 11 fixes this with APP_PREVIOUS_KEYS
: stores past keys to decrypt old data and maintain sessions during key rotation.
Laravel Reverb
Laravel Reverb is a high-speed real-time communication tool for Laravel apps. It integrates with Laravel’s broadcasting features and scales well by distributing traffic across multiple servers using Redis. This allows a single application to handle a large number of users with real-time updates.
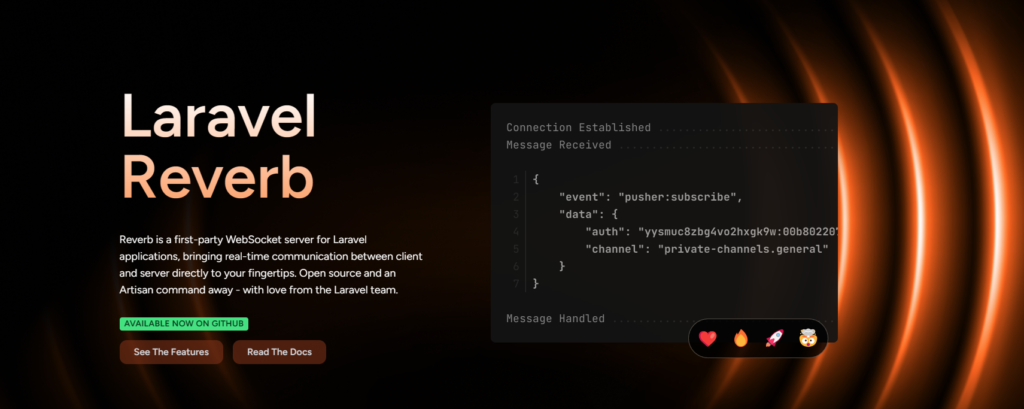
Please follow the Laravel 11 Release notes for in-depth details.
Following are some of the articles that will help you with Laravel
- Laravel Eloquent: Integrating CASE WHEN Statements with raw() for Advanced Querying
- Laravel Eloquent: “get()” vs “find()”
- Laravel : Integrating Social Media Share Buttons
- A Comprehensive Guide to Encryption and Password Hashing in Laravel 10
- Maximizing Laravel Performance with Caching: 4 Real-Life Problem-Solving